Git allows you to create branches, but it doesn’t tell you how to use them. In this article, let's dive into the most popular branching workflow and learn how to successfully identify base branches. We will also figure out how to perform branch comparisons, so that we can better understand what changes were made exclusively to a specific branch.
Branches are a vital part of Git. While there are several branching workflows that can be adopted, the "Git Feature Branch Workflow" is the most popular one. It assumes there is a central repository (usually by the name of main
, formerly master
) and developers are encouraged to create a new branch whenever it is time to work on a new feature.
These "Feature Branches" (sometimes called "Topic Branches") are key to this model. The main branch is left intact while developers work on different features in isolation. When the feature is ready, the feature branch is then merged back into the base branch.
This approach presents a couple of important advantages for feature development:
- Releases can be planned and managed in their own branches;
- Bugs can be detected early and fixed before reaching the
main
branch.
These branches should be short-lived, with the sole goal of providing an environment to work on a new feature. It is recommended that you merge often and early, deleting the branch once integration is complete.
This is a recommended best practice for continuous integration (CI). A long-lived feature branch, on the other hand, will increase the chances of having merge conflicts to solve.
One important aspect of this workflow revolves around Pull Requests. PRs, as they are also called, promote discussions among teams. Features can be approved, suggestions can be made, and conversations can take place, all before the branch gets integrated into the main branch. Pull Requests are also feature branches, they just contain some additional meta-information (such as comments and code reviews) on top.
The branch that a feature branch was originally branched off is called the "base branch". At some point, you may need to determine:
- Which was the branch that originated this feature branch;
- How many exclusive commits were added to the feature branch.
How would we go about it? Let's find out!
Not a Tower user yet?
Download our 30-day free trial and experience a better way to work with Git!
Locating the Base Branch That Originated the Feature Branch
There are two solutions worth looking into. One involves using git reflog
, while the other comprises finding the nearest merge base.
Option 1: Using git reflog
The base branch of a feature branch cannot be reliably determined automatically. To understand why, let's start by opening the command line.
We will create a new branch from main
and then investigate the reflog:
$ git branch feature/a main
$ git reflog feature/a
1cf3fde2d9 (HEAD -> main, feature/a) feature/a@{0}: branch: Created from main
We can see that "git reflog" creates an entry, but this information might not be available if:
- the reference log history has been cleaned up;
- someone else has created the feature branch on their machine and you joined work on that branch.
When this is the case, the next solution comes in handy.
Option 2: Finding the Nearest Merge Base
To guess the base branch in the terminal, you can run a script to find the nearest merge base.
Start by copying and pasting the code below to a script.sh
file. Then, make it executable by typing chmod +x script.sh
and finally run it by typing: sh script.sh [BRANCH]
(replacing [BRANCH] with the name of your feature branch).
If you'd like to run this command from anywhere, you can add that script to the /usr/local/bin
folder. This will also allow any other users on the system to run it.
#!/bin/sh
FEATURE_BRANCH=$1
if [ -z "$FEATURE_BRANCH" ]; then
echo "Usage: git-base-branches FEATURE_BRANCH"
exit 128
fi
NEAREST_MERGE_BASE=$(git merge-base $FEATURE_BRANCH $(git for-each-ref --format='%(refname:short)' refs/heads/ | grep -v $FEATURE_BRANCH))
git branch --contains $NEAREST_MERGE_BASE | grep -v $FEATURE_BRANCH | sed 's/^[[:space:]\*]*//g'
Now that we know the base branch, let's see how we can compare branches.
Figuring Out Which Commits Were Added to the Feature Branch
While working on a feature branch, you will often want to see what commits have been made on that specific branch to get a better view of what has actually changed. This is especially true if the branch hasn't been checked out for some time.
You might also want to go over the accumulated set of modified files and their changes. These needs are particularly relevant when going through a Pull Request.
To filter the commits that are exclusive to the feature branch (or vice-versa), you can type one of these two commands:
# 1. Shows new commits on feature branch compared to base
❯ git log --oneline BASE..FEATURE_BRANCH
# 2. Shows new commits on base compared to feature branch
❯ git log --oneline FEATURE_BRANCH..BASE
If we ran the first command, this is what we would get:
❯ git log --oneline main..feature/login
6bcf266 (HEAD -> feature/login, origin/feature/login) Optimize markup structure in index page
2b504be (origin/staging) Change headlines for about and imprint
0023cdd Add simple robots.txt
For the second command, the output would look a little different:
❯ git log --oneline main..feature/login
af0d627 (main) Optimize markup structure in index page
19ba654 Add simple robots.txt
71507e0 Change headlines for about and imprint
Keep in mind that while you work on a feature branch, more commits may be added to the base branch. The base branch will then get "ahead" of the feature branch.
If you know it will take you a while to complete that feature, it may make sense to merge the base branch back into the feature branch every so often, so that you can reduce the number of merge conflicts you will have to inevitably solve upon integration. By doing so, these should become easier to resolve.
You can also rebase a feature branch onto the latest version of the base branch before merging it back. Please be aware that this will change history, so this action should be avoided if you're working on a feature branch with other teammates. In certain cases, it can even break Pull Requests (e.g. active reviews of certain revisions).
Once your feature is finished and your work is complete, you will want to merge it back into the base branch (which is the same as closing a PR). At this point, you can also safely choose to rebase and merge the feature branch, as there won’t be any more changes.
You can then delete the branch and move on to another feature!
Branch Management Made Easy
Download our 30-day free trial and experience a better way to work with branches in Git!
Comparing Branches in Tower
As expected, branch comparison is a lot easier if you're using Tower, our Git client.
While viewing any branch's commit history, click the "Compare" icon to compare the feature branch against any other local branch. Tower will immediately filter out the commits that are exclusive to that feature branch.
In this example, we're pinpointing which new commits were added to the 17.0.2
branch by comparing it to the previous version (17.0.1
).
You can easily pick a different branch to compare. When clicked, the "Compare to" dropdown will present a list of local branches for you to choose from.
In this example, let's select main
as our base branch.
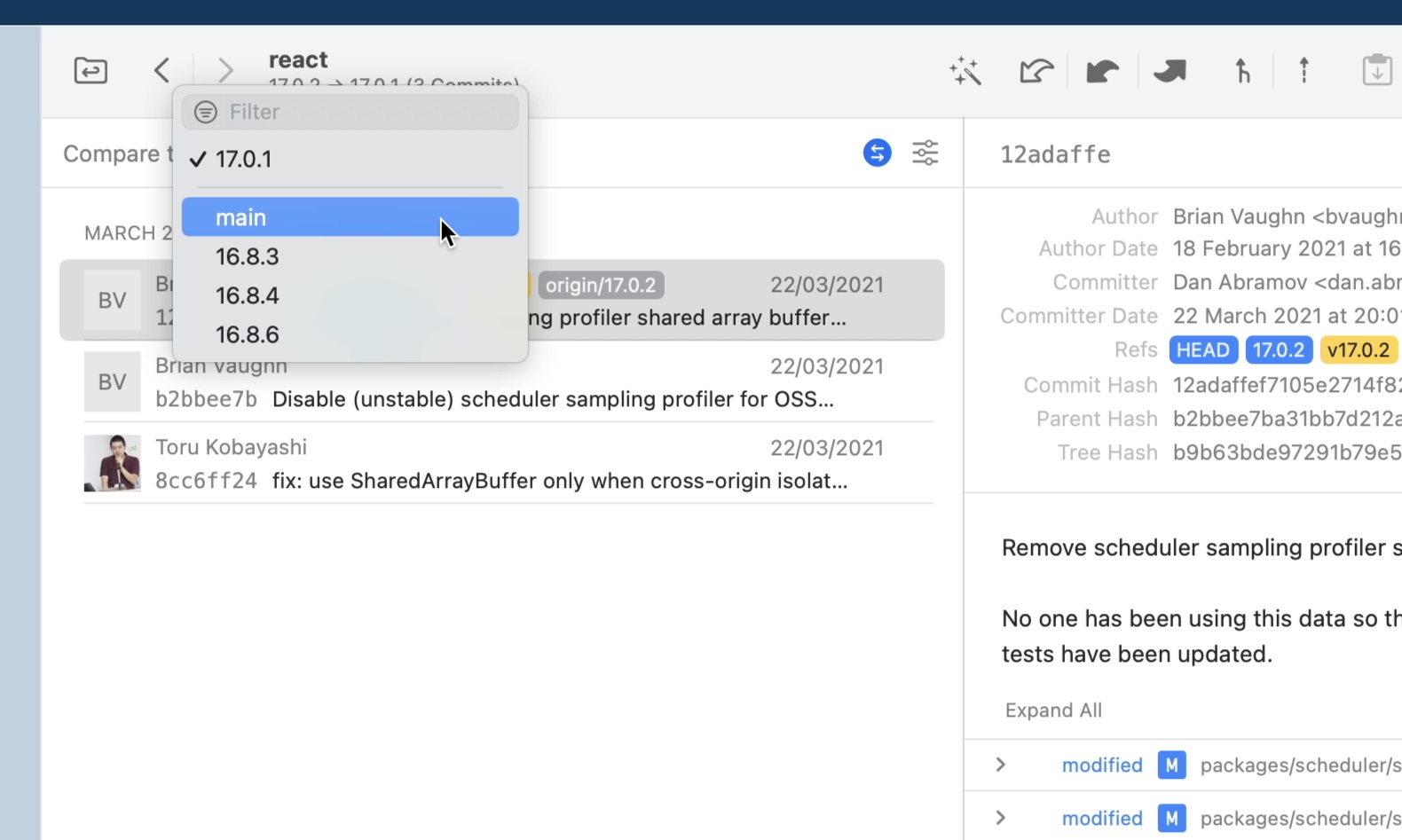
Depending on the branch you choose, you may be presented with additional information. An example would be the "Merge Conflict" warning.
In the image below, you can see that if we decided to merge 17.0.2
into main
, we would have to fix 18 merge conflicts.
You can click on the warning sign to identify the exact file names.
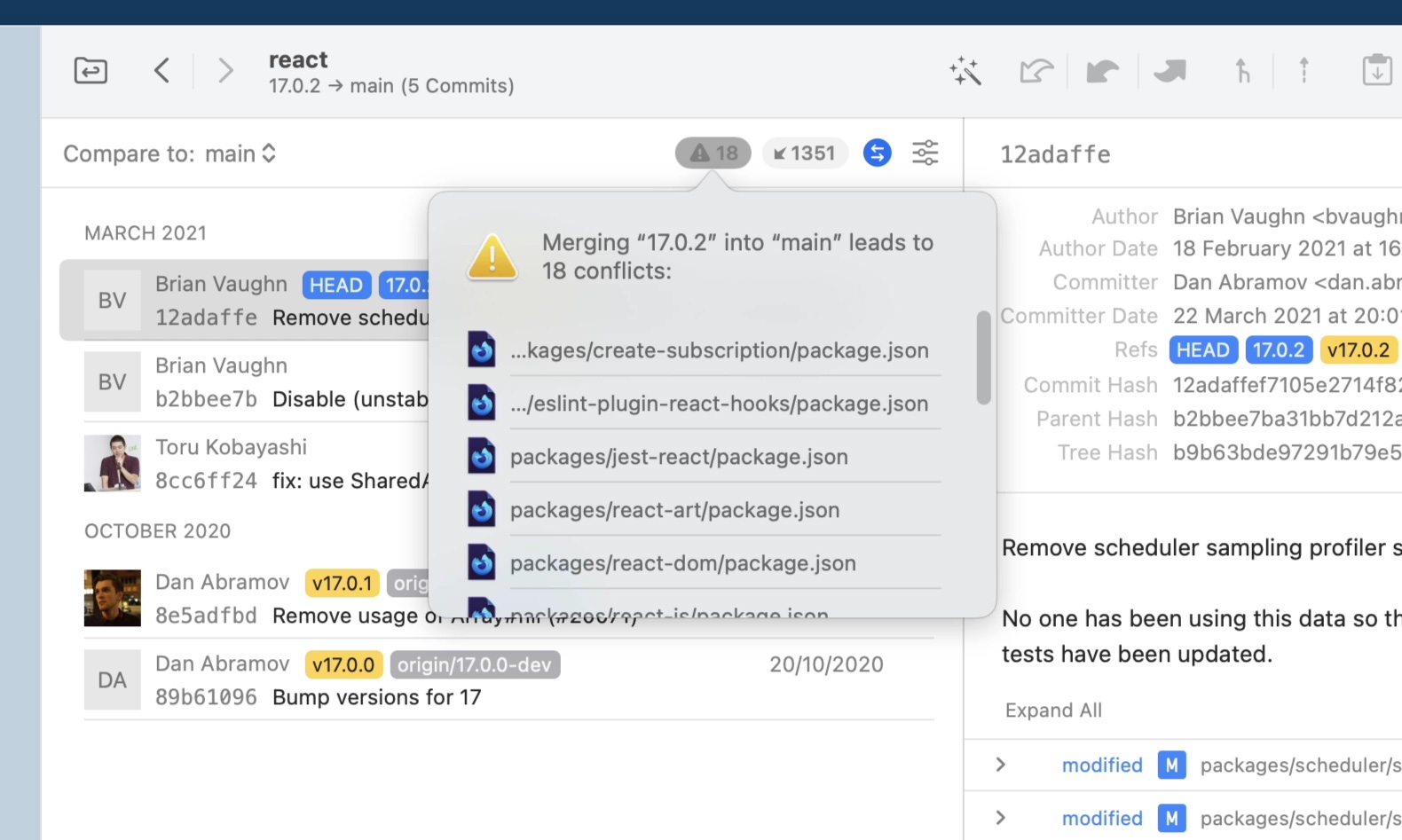
Next to it, you may see another notification. Tower will show the number of commits that the base branch is “ahead” of the feature branch.
In this example, 17.0.2
is 1351 commits behind main
.
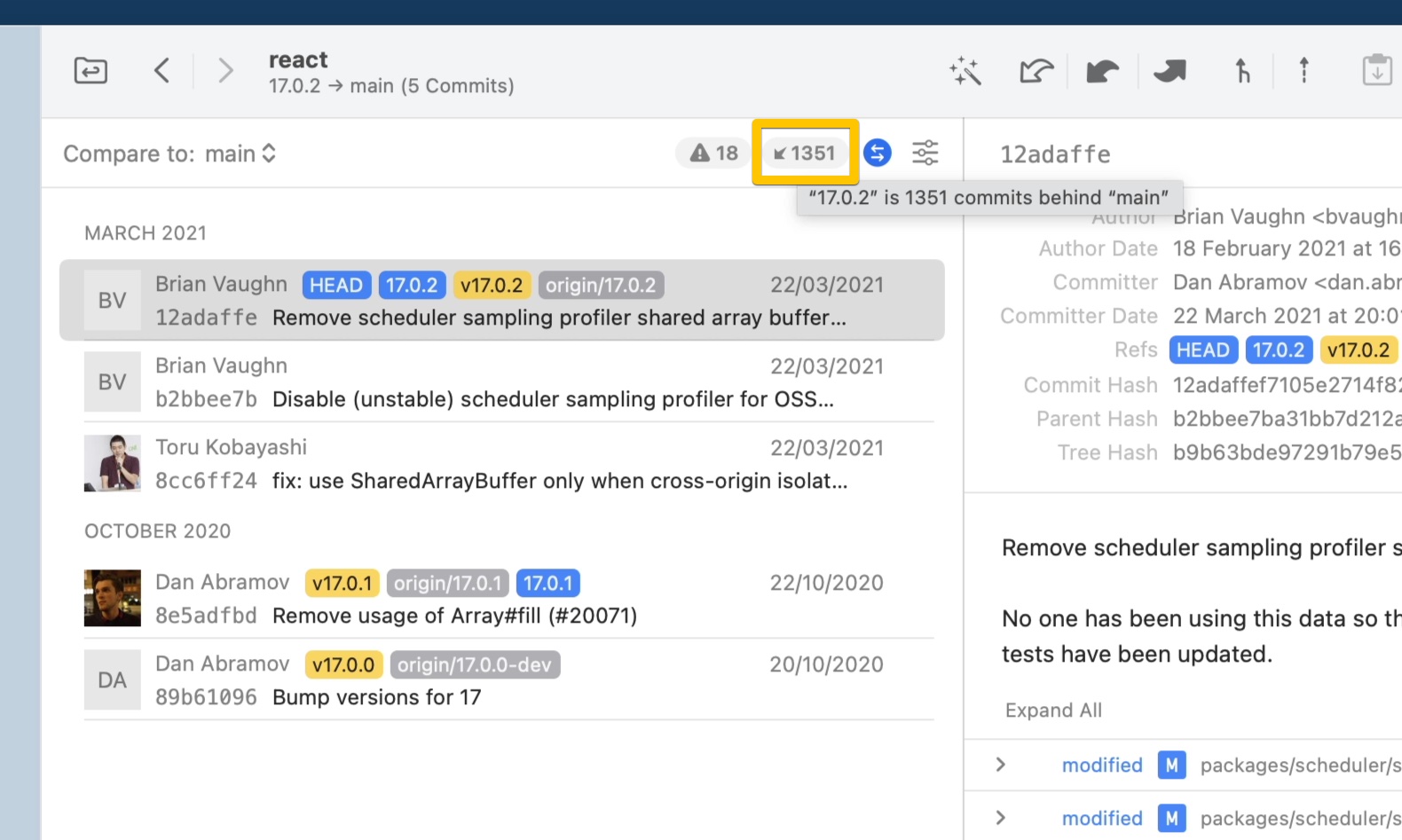
With the "Compare" toggle switched off, our Git client can detect any existing Pull Requests for this feature branch. Jumping to that specific PR is only a click away!
Branch comparison isn't the only branch-management feature that was added in Tower 8 for Mac. We have other aces up our sleeve, such as:
- Branch Review;
- Branch Pinning;
- Branch Filtering.
Make sure you read the Tower 8 for Mac announcement to learn how we implemented all these helpful tools.
If you're new to Tower, don't forget that you can try it for free for 30 days!